Creating a Chatbot in Python: A Comprehensive Guide
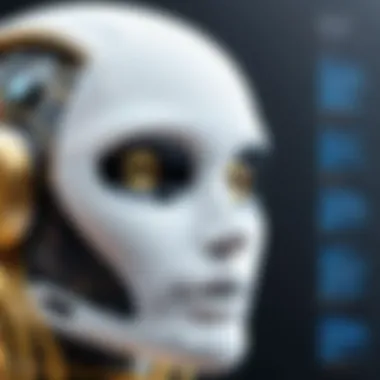
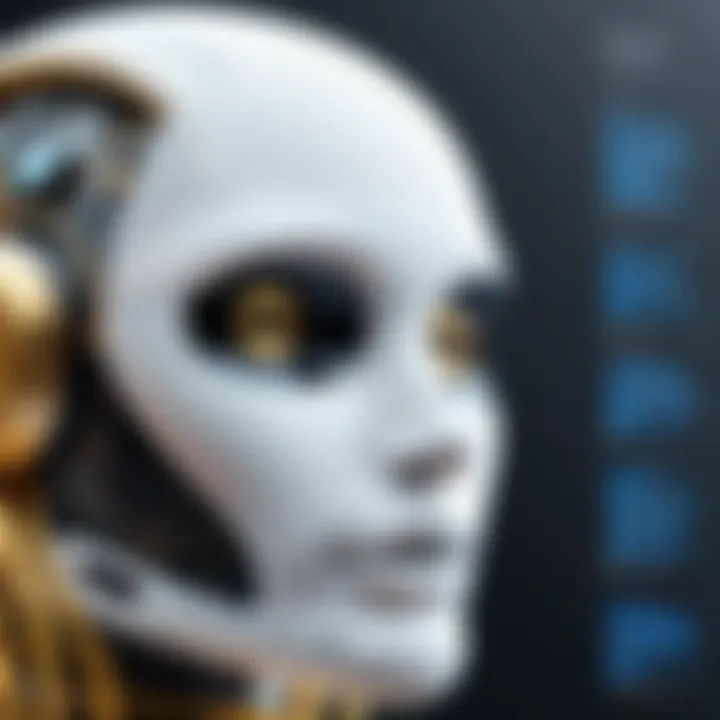
Intro
The development of chatbots has become a significant trend in the tech industry. Every year, more businesses and developers embrace the idea of conversational agents, making customer interactions smooth and intuitive. Utilizing Python for this task can enhance functionality thanks to its extensive libraries and easy-to-read syntax. Understanding how to create a chatbot with Python is essential for anyone looking to stay ahead in the ever-evolving world of technology.
This guide serves as a roadmap for both novice programmers and seasoned developers. It will elucidate the process of chatbot creation step-by-step, starting from foundational principles to advanced deployment techniques. With a focus on various frameworks, tools, and techniques, this narrative aims to equip readers with the knowledge required to develop and deploy a chatbot effectively.
Product Overview
Chatbots are computer programs designed to simulate conversation with human users. They have found applications in numerous areas, from customer service to personal assistants. This section describes the essential components of creating an effective chatbot using Python.
Description of the product
A chatbot in Python is essentially a software application that interacts with users via text or voice. It leverages natural language processing (NLP) to understand and respond to user queries in a meaningful way. The chatbot can either be rule-based, relying on predefined scripts, or AI-based, learning from interactions to provide improved responses over time.
Key features and specifications
- Natural Language Understanding: Ability to comprehend user input and interpret context.
- Machine Learning: Enhances response accuracy as more data gets processed.
- Integration Options: Capable of connecting with various platforms, such as Facebook Messenger, Slack, and WhatsApp.
- User-Centric Design: Focused on creating a smooth interaction experience with minimal friction.
- Scalability: Designed to handle a growing number of user interactions effectively.
In-Depth Review
Understanding the intricacies of chatbot development involves dissecting its performance and usability. This section will briefly examine these two critical aspects.
Performance analysis
The performance of a chatbot largely determines its usefulness. A well-optimized chatbot should be able to process queries quickly and return accurate answers. Key performance indicators include:
- Response Time: The time taken to understand and respond to user inputs.
- Accuracy Rate: Percentage of correct responses generated.
- Failure Rate: Instances where the chatbot fails to understand user requests.
By evaluating these metrics, developers can identify areas of improvement and refine their chatbots effectively.
Usability evaluation
Usability is crucial for the success of any chatbot. A user-friendly interface makes it easier for users to engage. Consider the following aspects when evaluating usability:
- Ease of Use: The interaction process should be straightforward.
- Feedback Mechanism: Allow users to provide feedback on responses to contribute to learning.
- Adaptability: The chatbot should evolve based on user needs and preferences.
Regular testing and updates can significantly enhance user experience.
The journey of creating a chatbot is not just about writing code; it involves understanding human interaction patterns.
As we proceed through this guide, expect to delve into various tools and frameworks that can assist in actualizing a chatbot project successfully.
Prelims to Chatbots
Chatbots have transformed how we interact with technology, acting as virtual agents that enhance user experiences across various platforms. Understanding the fundamental aspects of chatbots is crucial for anyone looking to design and implement these intelligent systems. This section delves into essential elements, benefits, and important considerations related to chatbots.
Definition of Chatbots
Chatbots are software applications designed to simulate human conversation. They utilize natural language processing (NLP) to interpret user inputs and provide relevant responses. Chatbots can function through text or voice interfaces, making them versatile tools for improving communication. They are programmed to recognize specific keywords or phrases, enabling them to understand user intents and deliver appropriate answers. In essence, chatbots serve as automated assistants that can manage queries efficiently.
History of Chatbot Development
The history of chatbots can be traced back to the 1960s with the creation of ELIZA, a program that mimicked human conversation through text. Subsequently, other models emerged, such as ALICE and Jabberwacky, which built on ELIZA's foundation but offered more advanced functionalities. The development of sophisticated algorithms and greater computational power in recent decades led to the emergence of context-aware and more interactive chatbots. Today, platforms like Google Dialogflow and Microsoft Bot Framework allow developers to create complex chatbots rapidly, revolutionizing how businesses engage with users.
Applications of Chatbots
Chatbots find applications across various sectors, offering numerous benefits:
- Customer Support: They assist in answering frequently asked questions and resolving common issues, freeing human agents for more complex tasks.
- E-Commerce: Chatbots can provide personalized recommendations based on user preferences, thereby enhancing shopping experiences.
- Healthcare: They play a role in patient scheduling, symptom checking, and providing basic health information.
- Education: Chatbots can facilitate learning by providing tutoring assistance or answering students' queries promptly.
Given the increasing reliance on digital communication, the role of chatbots is likely to expand further, making it essential to understand their capabilities and design principles.
Python as a Programming Language for Chatbots
Python has become a go-to language for chatbot development, primarily due to its versatility and ease of use. The language supports various programming paradigms, allowing developers to design chatbots that are both simple and complex. Additionally, Python's syntax is clear and intuitive, making it suitable for both beginner programmers and seasoned developers. This accessibility translates into faster development cycles.
Another critical factor is the abundance of libraries available for Python. These libraries simplify tasks such as natural language processing and machine learning—two essential components in creating intelligent chatbots. With Python, developers can easily integrate advanced capabilities, enhancing the chatbot's functionality without extensive coding from scratch.
Considerations while using Python involve its performance. Although generally adequate for chatbot interactions, Python is not as fast as some compiled languages. Yet, the trade-off often favors development speed and ease of use over execution speed, especially in prototype phases.
Python tends to simplify complex tasks, enabling rapid iterations on chatbot functionality.
Advantages of Using Python
One of the primary advantages of Python is its simplicity. The syntax is straightforward, which means developers can focus more on logic and less on complex code structures. This is particularly useful for rapid prototyping, allowing teams to test ideas and iterate quickly.
Python also has a large, active community. This means that developers can easily find support and resources. Forums like Reddit provide platforms for exchanging ideas and troubleshooting issues, making Python development a collaborative effort.
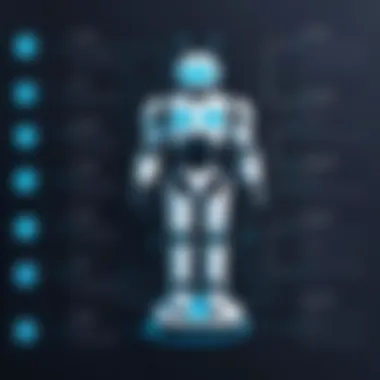
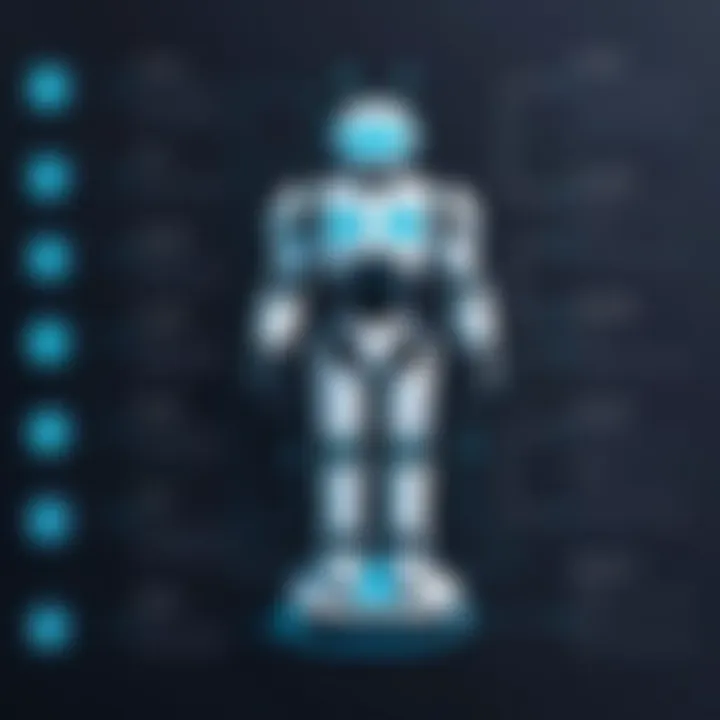
Some specific benefits include:
- Wide Adoption: Python is widely used across various domains, increasing the likelihood that developers will find familiar tools.
- Rich Libraries: Libraries such as NLTK, SpaCy, and TensorFlow provide powerful tools for implementing natural language processing and machine learning.
- Cross-Platform Compatibility: Python applications are platform-agnostic, allowing chatbots to run on various systems without additional modifications.
Relevant Python Libraries and Frameworks
For anyone looking to build a chatbot in Python, several libraries and frameworks stand out. These include but are not limited to:
- NLTK: The Natural Language Toolkit is particularly useful for tasks related to linguistic data, making it easier to parse and understand user input.
- SpaCy: This library is optimized for performance and is noted for its efficient tagging and parsing capabilities.
- ChatterBot: Specifically designed for training conversational agents, ChatterBot can learn from the conversations it processes.
- Flask and Django: Both are web frameworks used to create web applications, and they can be used to develop back-ends for chatbots, handling user requests and responses seamlessly.
These tools and libraries simplify techniques such as handling user input, processing language, and generating appropriate responses, making Python a robust option for developing chatbots.
By choosing Python as the foundation for building chatbots, developers unlock a plethora of resources that streamline the development process while enhancing the application's functionality. With its clarity, community support, and powerful libraries, Python remains a pivotal player in the chatbot development landscape.
Setting Up the Development Environment
Setting up the development environment is a crucial step in creating an efficient and functional chatbot. A well-prepared environment not only streamlines the development process but also ensures that all dependencies are managed properly. This section will discuss two main areas: installing Python and its dependencies, as well as choosing an integrated development environment (IDE). Each step contributes significantly to the chatbot development workflow, enhancing productivity and minimizing potential issues down the line.
Installing Python and Dependencies
Installing Python is the first and foremost task in setting up your development environment. Python is renowned for its simplicity and readability, making it an ideal choice for novice and experienced developers alike. The installation process is straightforward, whether you're using Windows, Mac, or Linux systems. Here’s a simplified breakdown of the steps:
- Download Python: Access the official Python website (https://www.python.org/) and download the latest version.
- Run the Installer: Execute the installer and ensure you tick the option that adds Python to the system path. This step is vital for command line access.
- Verify Installation: Open a command prompt or terminal and type to confirm the installation. If the version number appears, you’re set.
Once Python is installed, managing dependencies is the next task. Using a package manager like pip allows easy integration of additional libraries like NLTK or TensorFlow, which are essential for building chatbot functionalities. Simply use the command:
This command will fetch and install the desired library directly. Keeping dependencies up to date using commands such as helps maintain an optimal coding environment.
Choosing an IDE
The next significant step involves selecting an Integrated Development Environment (IDE) that aligns with your workflow. An IDE provides a comprehensive platform for writing code, debugging, and managing projects. The choice of IDE can greatly influence productivity and comfort during development. Several options stand out:
- PyCharm: This is a robust IDE tailored specifically for Python. It offers powerful features such as code completion, debugging tools, and version control integration.
- Visual Studio Code: A versatile and lightweight code editor which supports Python through extensions. Its customization capabilities make it popular among many developers.
- Jupyter Notebook: Ideal for those who prefer an interactive coding experience. It allows you to write code in sections and see instant results, which is especially useful for experimentation.
When choosing an IDE, consider factors like user interface, features, and community support. Testing a few options may lead to a better fit for your needs.
"The right tools can significantly enhance your development experience and efficiency."
By thoughtfully setting up your development environment, you establish a solid foundation for creating your chatbot. The initial time investment in configuring Python and selecting an IDE can yield long-term benefits, allowing for a smoother and more enjoyable development journey.
Core Components of a Chatbot
The development of an effective chatbot hinges on its core components. Each element plays a crucial role in how well the chatbot interacts with users. By understanding these components, developers can create more engaging and intelligent conversational agents. There are several factors to consider, notably user input handling, natural language processing techniques, and response generation strategies.
User Input Handling
User input handling is fundamental to the chatbot's operation. This component focuses on capturing and processing the input provided by users. It should be designed to accommodate various types of data, including text, voice commands, and even emojis. A well-structured input handling system ensures that the chatbot can recognize and manage different user intents effectively.
For instance, if a user types a question, the system should be able to extract relevant keywords and context to generate an accurate reply. This often involves parsing the input and using techniques like keyword extraction or intent recognition. An effective user input handling mechanism increases user satisfaction by making the interaction smoother and more intuitive.
Natural Language Processing Techniques
Natural language processing (NLP) techniques are at the heart of any chatbot’s ability to understand and generate human language. These techniques allow chatbots to interpret user inputs in a way that resembles human conversation. Some key NLP techniques include tokenization, stemming, and named entity recognition.
- Tokenization: This process breaks down input text into individual words or phrases, allowing the chatbot to analyze it more easily.
- Stemming: This technique reduces words to their base or root form, making it easier for the chatbot to understand variations in user input.
- Named Entity Recognition (NER): This helps the bot identify specific entities such as dates, names, or locations from user inputs.
By leveraging these techniques, chatbots can achieve a higher level of accuracy in understanding queries and providing relevant responses. The right application of NLP can significantly enhance the chatbot's effectiveness and user experience.
Response Generation Strategies
After processing user inputs, the chatbot must generate appropriate responses. This component is vital to how meaningful and helpful interactions become. A few common strategies for generating responses include rule-based systems, retrieval-based systems, and generative models.
- Rule-based systems utilize predefined rules and scripts to provide responses based on specific keywords or phrases.
- Retrieval-based systems search through a database of responses to find the most suitable one.
- Generative models employ machine learning techniques to create responses from scratch, making them more adaptable and dynamic.
Each strategy has its own strengths and weaknesses. Choosing the right one depends on the desired complexity and flexibility of the chatbot. Generally, combining multiple strategies can yield better results, allowing for more nuanced conversations.
In summary, the core components of a chatbot represent essential building blocks that determine the system's efficiency and effectiveness in user interaction. Understanding these components deeply aids developers in creating better conversational interfaces.
Training Your Chatbot
The effectiveness of a chatbot heavily relies on its training. When we talk about training your chatbot, we refer to the process of improving its ability to understand and respond appropriately to user input. This is essential because a well-trained chatbot can significantly enhance user experience, leading to greater engagement and satisfaction. The importance of this phase cannot be understated, as it lays the groundwork for how your chatbot will perform in real-world scenarios.
To train your chatbot effectively, you need to focus on the two main aspects: data collection and the training methodologies you choose. Each of these elements plays a critical role in shaping the chatbot's responses and ensuring it adapts to various user needs and language styles.
Data Collection for Training
Data collection is the first step in training a chatbot. Good quality data ensures that your chatbot learns the right patterns and responses. There are several sources from which you can gather data. These include:
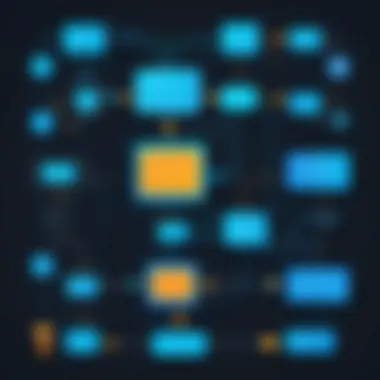
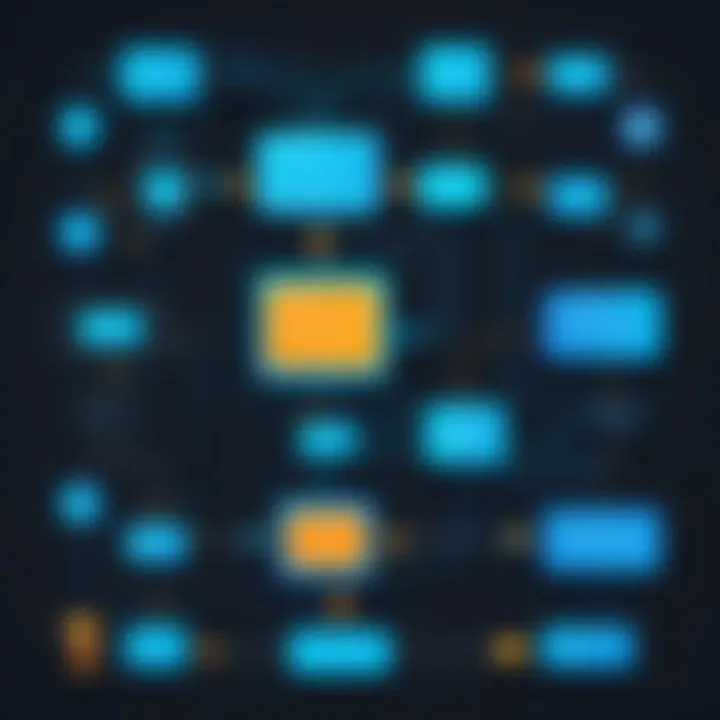
- Existing Chat Logs: If you have previously deployed chatbots, analyze chat logs. They provide real-world examples of how users interact.
- Surveys and Feedback: Gathering input from real users who identify what they expect from the chatbot can help define the data you need.
- Open Datasets: Use publicly available datasets specifically designed for chatbot training. Websites like Wikipedia and Reddit have text corpora that can be beneficial.
The collected data should cover a variety of topics relevant to the expected queries. This diversity allows the chatbot to respond accurately to a broader array of conversations. It's also necessary to preprocess the data. Preprocessing might include cleaning the text, removing stop words, and normalizing the data.
Supervised vs. Unsupervised Learning
Choosing the right learning approach is fundamental in chatbot training. You have two primary methods to consider: supervised learning and unsupervised learning. Each has its pros and cons, and the choice depends on your training objectives.
- Supervised Learning: In this approach, the chatbot is trained using labeled data. For example, input data are paired with the correct responses. This method allows for precise learning since the expected output is clear. It is beneficial for tasks like intent recognition and entity extraction. However, one drawback is that it requires a significant amount of labeled data, which can be time-consuming to obtain.
- Unsupervised Learning: Here, the chatbot does not rely on labeled input. Instead, it identifies patterns and structures in the data autonomously. This method can be useful for generating conversation and understanding topics but may lead to variability in responses. The chatbot might not have a definitive guideline to learn from, making it less predictable.
Ultimately, combining both supervised and unsupervised learning strategies can yield the best results. This approach maximizes the strengths of both methodologies, allowing for a more robust training process.
"Effective training is crucial for enhancing the performance and adaptability of chatbots in various user scenarios."
Integrating Machine Learning
Integrating machine learning enhances the capability of chatbots significantly. This integration allows chatbots to learn from user inputs, customize responses, and adapt over time. By utilizing machine learning algorithms, a chatbot can provide a more personalized experience for users. This advanced functionality can improve user satisfaction and retention rates, which are key metrics for any chatbot's success.
When discussing integrating machine learning in chatbot development, several critical considerations arise. Developers must choose the right machine learning model based on the specific use case of their chatbot. For instance, a customer service chatbot might benefit from a supervised learning model to train on historical interactions, while a personal assistant may utilize unsupervised learning to discover user preferences.
Moreover, scalability is an essential aspect. As the chatbot interacts with more users, its training data will expand. This requires a flexible architecture that can grow without compromising performance. Therefore, strategies to retrain the model or update the data pipeline should be part of the initial design process.
The following sections will outline the necessary libraries for implementing machine learning in chatbots and how to develop intelligent systems that leverage AI's strengths for enhanced interactions.
Machine Learning Libraries for Chatbots
A variety of machine learning libraries are available to facilitate the creation of chatbots in Python. Here are some popular options:
- TensorFlow: A comprehensive library for building machine learning models. TensorFlow offers extensive documentation and community support, which is valuable for both beginners and experts.
- Keras: Useful for rapid prototyping of deep learning models. It operates on top of TensorFlow, making it user-friendly while still powerful.
- scikit-learn: This library is ideal for traditional machine learning algorithms and is simple to integrate with existing projects.
- spaCy: A library specifically aimed at natural language processing (NLP) tasks, offering pre-trained models that can be utilized for analyzing user input effectively.
- NLTK: The Natural Language Toolkit is excellent for working with human language data, providing tools that facilitate text processing and linguistics manipulation.
Each library has its strengths, and the choice largely depends on the chatbot's requirements. For instance, if a chatbot primarily focuses on language understanding, spaCy or NLTK would be more suitable.
Building a Smart Chatbot with AI
To create a smart chatbot, several considerations are fundamental. First, focus on the chatbot's learning capabilities. Implementing reinforcement learning can help the bot learn from interactions, rewarding it for providing accurate responses and penalizing incorrect ones. This technique not only improves response accuracy over time but also enhances user interaction quality.
Next, the chatbot should have access to a wide range of data. This data acts as the foundational knowledge base. Chatbots that rely on comprehensive datasets can offer better insights and improved service. Regular updates to the dataset are necessary to maintain relevance with current user trends and preferences.
It is also essential to incorporate sentiment analysis. Understanding user emotions can vastly improve a chatbot's response effectiveness. Utilizing libraries that facilitate sentiment analysis can aid in creating responses that are not just contextually relevant but also emotionally appropriate.
"A well-constructed chatbot can unlock new dimensions of user interaction, making technology feel more intuitive and responsive."
In summary, integrating machine learning into a chatbot not only makes it smarter but also enhances user engagement and satisfaction. By carefully selecting the right libraries and models, developers can build chatbots that effectively meet user needs and evolve with user interactions.
Testing Your Chatbot
Testing is a critical phase for any chatbot development process. Just like any software product, a chatbot must perform reliably and meet user expectations. The importance of testing your chatbot lies in its ability to enhance user experience, identify potential issues, and ensure that it functions correctly across various scenarios. In this section, we will delve into the essential aspects of testing, including scenarios and use cases, as well as the significance of gathering user feedback for continuous improvement.
Testing Scenarios and Use Cases
When testing a chatbot, it is crucial to establish diverse scenarios and use cases that reflect real-world interactions. This includes not only typical user questions but also edge cases that may reveal system limitations. A well-structured testing plan should include:
- Conversational Flow: Test various paths a conversation might take. Ensure that the chatbot can handle interruptions and return to the main topic gracefully.
- Response Accuracy: Examine the relevance and accuracy of responses to user queries. Use specific examples to verify that the chatbot's answers are correct.
- Error handling: Evaluate how the chatbot responds to unrecognized input or ambiguous questions. It should provide helpful prompts or steer the conversation in a more productive direction.
- Performance Under Load: Assess how the chatbot behaves when multiple users interact with it simultaneously. This is vital for understanding limits in scalability and performance.
Conducting tests across these areas allows developers to spot weaknesses early in the deployment cycle and refine the chatbot before it reaches the end-user. Proper testing can also help teams identify the specific problems with the design or implementation, thereby leading to more effective solutions.
User Feedback and Iterative Improvement
Collecting user feedback is essential for the iterative improvement of a chatbot. Once the initial deployment occurs, it is important to monitor interactions and gather insights from users. This feedback can guide developers on how well the chatbot meets user needs and where improvements can be made. Consider the following strategies to gather and implement user feedback effectively:
- Surveys and Polls: Directly ask users to rate their experience and provide comments after using the chatbot. This feedback can highlight both strengths and weaknesses.
- Analyzing Conversation Logs: Review conversations to identify patterns in user interactions. Understanding what users frequently ask can help refine the chatbot’s responses.
- Implement a Feedback Loop: Automatically prompt users for feedback after specific interactions or when the chatbot fails to understand a query. This encourages continuous communication between users and developers.
- Iterate Based on Insights: Use the collected data to make informed updates. It might include refining the chatbot's language model, enhancing its knowledge base, or improving the handling of specific queries.
"Continuous feedback is the backbone of iterative development, helping to mold a chatbot into a better conversational partner."
In summary, testing a chatbot involves careful consideration of various scenarios and incorporation of user feedback for improvement. Ensuring that the chatbot can handle a range of interactions effectively, coupled with an ongoing commitment to refining its abilities based on real-world use, is fundamental to a successful chatbot deployment.
Deployment of the Chatbot
Deploying a chatbot is a critical step in the development process. It defines how users will interact with the bot, determining its accessibility and functionality across different environments. The deployment phase solidifies the chatbot's purpose in real-world applications. This section dives into the essentials of deployment, focusing on key aspects like choosing a hosting platform and integrating with messaging platforms.
Choosing a Hosting Platform
Selecting the right hosting platform is vital for a successful chatbot deployment. Different platforms offer varying features, scalability options, and price points. Here are several factors to consider:
- Scalability: Consider how many users your chatbot will handle. Platforms like Heroku or AWS allow for easy scaling as user demand increases.
- Performance: Look for platforms that provide faster responses. The hosting solution shouldn't be a bottleneck in user experience.
- Support: Select a platform with good customer support. This is especially useful during initial deployment.
- Cost: Analyze the pricing model of different hosting services. Choose one that fits your budget while meeting all necessary requirements.
Some popular options include:
- Amazon Web Services (AWS)
- Microsoft Azure
- Google Cloud Platform
- Heroku
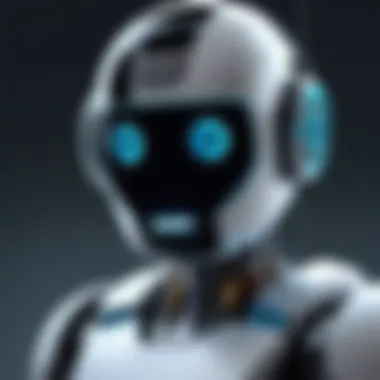
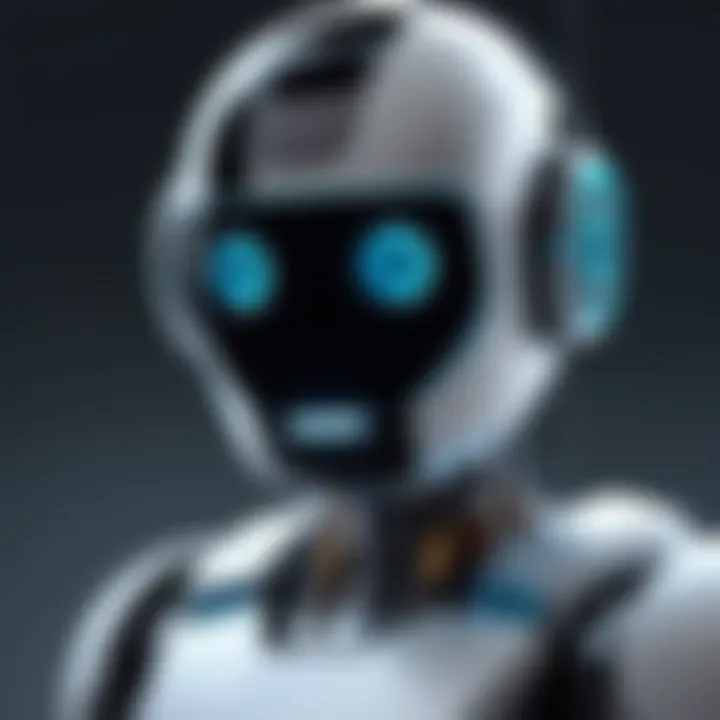
"Choosing the appropriate hosting platform can dictate the overall efficiency and effectiveness of your chatbot operation."
Integrating with Messaging Platforms
Integration with messaging platforms is equally significant. It dictates how users will engage with the chatbot and where it will operate. Popular messaging platforms include Facebook Messenger, Slack, and WhatsApp. Each platform has its own set of APIs and guidelines for integration.
When planning this integration, consider the following:
- User Experience: Ensure the chatbot's tone and style align with the messaging platform's user interaction norms.
- API Compatibility: Each platform has its APIs. Verify your chatbot can effectively communicate with those APIs.
- Security Features: Look into how the messaging platform addresses user security. Maintaining user privacy is paramount.
Once the chatbot is designed and tested, deploying it on selected messaging platforms makes it accessible to end-users. After integration, consider ongoing adjustments based on user interactions — this iterative process helps fine-tune the chatbot for better performance.
In sum, effective deployment strategies are essential for leveraging the full potential of your chatbot. Balancing hosting considerations with messaging integration opens up avenues for robust user engagement.
Maintenance and Continuous Improvement
In the realm of chatbot development, maintenance and continuous improvement are not merely optional; they are vital. Once a chatbot is deployed, it becomes essential to ensure that it operates efficiently and effectively. Regular maintenance helps to identify and rectify problems that may arise post-deployment. Continuous improvement, on the other hand, focuses on refining the chatbot's capabilities based on user interactions and feedback.
Monitoring Performance Metrics
Monitoring performance metrics is a critical aspect of maintaining a successful chatbot. It involves tracking various parameters that indicate how well the bot is performing. Key metrics include:
- User Engagement: Measuring the number of users interacting with the bot over time. A decrease may indicate a problem.
- Response Accuracy: Assessing how accurately the chatbot understands user input and delivers relevant responses. High accuracy is crucial for user satisfaction.
- Response Time: Analyzing the time taken by the bot to provide responses. Faster response times generally lead to better user experience.
Establishing baseline metrics at the time of deployment allows developers to gauge performance over time. Tools such as Google Analytics or specific chatbot analytics platforms can be employed for this purpose. By continuously monitoring these metrics, developers can pinpoint areas needing enhancement and address them proactively.
Updating Chatbot Capabilities
Updating the capabilities of a chatbot is essential for maintaining its relevance. As user needs evolve, so should the chatbot. Updates may include:
- Adding New Features: Incorporating new functionalities based on user feedback or advancements in technology. This ensures that the chatbot remains competitive and useful.
- Improving Natural Language Understanding: Regular updates to the natural language processing model can enhance the bot's ability to interpret user queries more effectively, thus improving user interaction.
- Integrating User Feedback: User feedback should be systematically gathered and analyzed. It can highlight common pain points which can then direct future enhancements.
A well-structured update cycle helps in keeping the chatbot aligned with user expectations and technological advancements. By promoting an environment of continuous improvement, developers can ensure that the chatbot does not stagnate but instead evolves to meet the demands of its audience.
Ethical Considerations in Chatbot Development
The development of chatbots brings with it a set of ethical considerations that are critical for creators to address. As chatbots become more prevalent in user interactions, understanding ethical implications is essential. It helps ensure that the technology is used responsibly and respects the rights of users. This section delves into two main aspects: data privacy concerns and bias in AI models. These considerations are vital for fostering trust and safeguarding the integrity of chatbot applications.
Data Privacy Concerns
Data privacy is a significant issue in chatbot development, primarily because chatbots often handle personal user information. Users engage with chatbots for various services, from customer support to personal assistance. During these interactions, bots may collect sensitive data, such as names, addresses, and preferences.
Developers must implement robust data protection measures. This includes ensuring secure data transmission and storage. Chatbots should adhere to privacy regulations like the General Data Protection Regulation (GDPR). With GDPR, users have the right to understand how their data is used. Periodically reviewing privacy policies and making them accessible to users is crucial. Here are some key practices in addressing data privacy concerns:
- Transparency: Inform users about what data is being collected and how it will be used.
- Consent: Obtain explicit user consent before collecting data.
- Limit Data Collection: Only gather information that is necessary for the chatbot's function.
- Secure Storage: Use encryption and security protocols to protect stored data.
By prioritizing data privacy, developers can enhance user trust and promote safer interactions with chatbots. More importantly, these practices can help prevent potential legal issues stemming from data misuse.
Bias and Fairness in AI Models
Bias is an inherent challenge in developing AI models, including those used in chatbots. AI systems learn from data, often reflecting the prejudices present in that data. If not properly managed, this can lead to unfair outcomes that disproportionately affect certain user groups. For instance, a chatbot designed for customer service might respond differently based on the linguistic or cultural background of a user.
To mitigate bias, it is essential to evaluate the datasets used in training chatbots. Developers should strive to use diverse datasets that represent a wide range of demographics and perspectives. Continuous testing for bias throughout the chatbot's lifecycle can identify unintended discriminatory behaviors.
Key strategies to address bias in AI models include:
- Diverse Training Data: Use datasets that are representative of all user groups.
- Bias Audits: Conduct regular assessments to identify and correct biases in chatbot responses.
- User Feedback: Incorporate input from users regarding their experiences and perceptions.
Fairness in AI is non-negotiable if chatbots are to serve a diverse user base effectively. Developers who focus on creating fair and equitable chatbots not only comply with ethical standards but also increase their potential user base.
"Ethics in AI development is paramount, as it shapes the user experience and public perception of technology."
The Future of Chatbots
The trajectory of chatbot development is a critical area of exploration, particularly as technologies continue to advance at an unprecedented pace. Chatbots are far more than just simple response-generating tools; they are evolving into complex systems capable of genuine interaction and learning. As this article illustrates, understanding the future of chatbots is essential, not only to grasp upcoming trends but also to anticipate the changes that may impact various industries and user experiences.
Emerging Trends in Chatbot Technology
Current advancements in artificial intelligence shape the future of chatbots significantly. These emerging trends are starting to redefine how organizations implement and leverage chatbot functionality.
- Conversational Commerce: Businesses are integrating chatbots into their e-commerce platforms. This allows for real-time customer assistance, aiding users through purchase decisions seamlessly.
- Voice Assistants: With the rise of voice recognition technologies, more chatbots are adapting to support voice interactions. This offers a natural interface that aligns closely with user behavior.
- Multi-Modal Interfaces: The focus is gradually shifting towards creating interfaces that combine text, voice, and visual elements. This enhances user engagement and leads to richer interactions.
- Integration with IoT: As smart devices proliferate, chatbots are finding new applications within the Internet of Things ecosystem, allowing users to control their smart environments via conversational interfaces.
These trends underline the necessity for developers and organizations to stay ahead of the curve, ensuring their chatbots remain relevant in marketplace.
Potential Advancements in AI Capabilities
The prospective developments in AI capabilities are set to transform chatbot functionalities further. Here are some crucial advancements to watch for:
- Natural Language Understanding: Enhanced NLP will enable chatbots to better comprehend user intent and context. This improvement means more accurate and meaningful conversations.
- Personalization: Future bots will likely leverage user data to offer tailored interactions. This significantly enhances the user experience by making interactions feel more relevant and thoughtful.
- Emotion Detection: With advancement, chatbots may evolve to recognize emotional cues in users, allowing them to respond with appropriate empathy or enthusiasm.
- Autonomous Learning: Future chatbots will be equipped with machine learning models that allow them to improve and adapt based on user interactions without constant retraining.
These advancements indicate that the future of chatbots is poised to be not only smarter but also more in tune with the needs and emotions of users.
"The future of chatbots isn't merely in improving communication, but in enhancing understanding and emotional relevance."
As we anticipate these changes, it is crucial for developers and businesses to remain adaptable. By doing so, they will ensure their chatbot solutions are both effective and meaningful moving forward.