Create and Add Your Own Custom Discord Bot
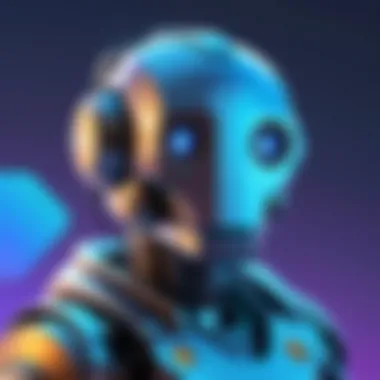
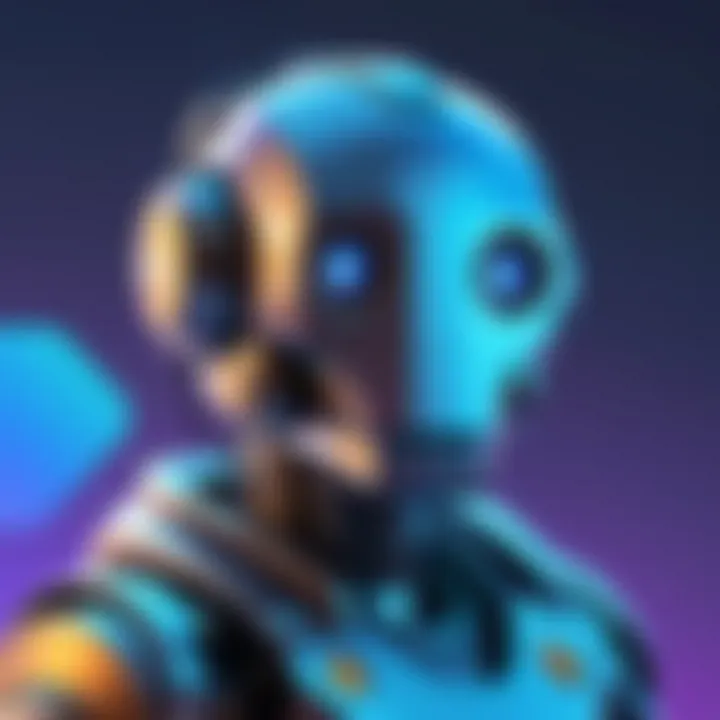
Intro
In the fast-evolving digital landscape, customizing platforms to fit personal preferences fosters creativity and enhances user experiences. Discord stands out in this aspect, providing a unique opportunity for users to develop and implement their own bots. A bot can automate tasks, manage roles, or even engage your community with tailored interactions. This guide will elucidate the entire process of creating and integrating a Discord bot into your server, regardless of your technical background.
Embarking on this journey involves understanding several key components. First, you will learn about the basic functionalities and significance of Discord bots. From there, we will explore programming languages that are suitable for bot development, delve into frameworks, and move onto deployment strategies. Additional layers of management and maintenance will also be discussed to ensure your bot runs smoothly over time. Understanding this multifaceted approach can elevate user engagement and streamline server management.
This narrative is designed to resonate particularly with tech-savvy individuals, gadget lovers, and early technology adopters. Through this comprehensive guide, not only will readers gain the practical knowledge needed to build a bot, but also acquire insights into best practices that foster efficient bot performance.
Prologue to Discord Bots
In the realm of online communication, Discord stands out as a platform that foster communities through its unique features. At the heart of many Discord servers are bots. These automated programs enhance user experience and streamline various tasks. This section explores the significance of Discord bots and sets the stage for your journey into bot development.
What is a Discord Bot?
A Discord bot is an application that runs automated tasks on a Discord server. These tasks can range from managing users to providing entertainment and information. Developers create bots using Discord's API (Application Programming Interface). This allows them to interact with various aspects of a server, such as sending messages, organizing channels, and integrating third-party apps. Bots can work without human intervention, which greatly increases the efficiency of managing online communities.
Some examples of common tasks performed by Discord bots include:
- Sending scheduled messages
- Moderating content based on set rules
- Playing music from various services
- Providing game stats and leaderboards
Bots essentially act as assistants, making it easier for server owners to maintain control and promote engagement.
Benefits of Using Discord Bots
The integration of Discord bots offers numerous advantages for users and administrators alike, including:
- Automated Tasks: By automating routine activities, bots save time and reduce the workload for moderators and server owners.
- Enhanced User Experience: Bots can introduce fun features like games, quizzes, and music, making a server more engaging for its members.
- Real-time Notifications: Bots can provide updates and alerts based on server events, ensuring that users stay informed.
- Advanced Moderation: Bots enhance moderation capabilities by detecting spam and inappropriate content, fostering a safer online environment.
- Customizability: Unlike static programs, bots can be tailored to match the specific needs and preferences of a server.
By incorporating a bot, you access a powerful tool for community management and engagement, transforming how members interact with each other.
Prerequisites for Bot Development
Before diving into the complex world of Discord bot development, it is essential to lay a solid foundation. Understanding the prerequisites will help streamline the process, leading to a more efficient development experience. By aligning your skills and tools, you can avoid common pitfalls and hasten your path to creating functional and engaging bots.
Understanding Discord's API
Discord's Application Programming Interface (API) is the backbone of any bot development process. This API allows developers to interact with Discord servers, users, and messages programmatically.
By familiarizing yourself with the API, you can unlock numerous possibilities for your bot's functionality. The API provides endpoints for sending messages, managing roles, and more. Here are critical points to note:
- Documentation: The official Discord API documentation is a valuable resource. Reading through it provides insight into how different endpoints work. This understanding is crucial for implementing various features.
- Requests and Responses: Knowing how to send requests and handle responses will form the crux of your interaction with the API. Understanding concepts like rate limits is vital for smooth operation.
- Libraries: Various libraries, such as Discord.js, can abstract some complexities. However, general knowledge of the API’s workings will improve your ability to troubleshoot and extend functionality.
Required Technical Skills
A successful Discord bot developer should possess certain technical skills. Although the authoritative list can differ based on personal preference, the following essentials can help build a solid foundation:
- JavaScript or Python Knowledge: Most Discord bots are written in JavaScript with Node.js or Python. Familiarity with one of these programming languages is crucial. You should understand syntax, data structures, and control flow, which will enable effective coding.
- Basic Web Development: Having a grasp of web technologies like HTML, CSS, and REST protocols will help understand how the backend and frontend communicate. This knowledge might come in handy, especially when adding advanced features to your bot.
- Debugging Skills: Bugs are inevitable when writing code. The ability to troubleshoot and debug is essential. You will need to diagnose errors and resolve them efficiently to ensure smooth functionality.
- Version Control with Git: Familiarity with version control systems like Git can help manage your code effectively. This ensures that you can track changes and collaborate with others if needed.
Understanding these prerequisites not only prepares you for the challenges ahead but can also enhance your overall ability in developing high-quality Discord bots. Being well-prepared will lead to a more seamless development process.
Setting Up the Development Environment
Setting up the development environment is critical in building a Discord bot. It lays the groundwork for coding and testing your bot in a controlled space. This process ensures that all necessary tools are installed correctly and that the environment is tailored for your specific bot requirements. A well-structured setup can save considerable time and effort later on.
Installing Necessary Tools
Node.js
Node.js is a runtime environment that allows you to execute JavaScript code on your server. It is widely recognized for its non-blocking I/O model, making it lightweight and efficient. This is especially valuable when building Discord bots, as they often handle multiple connections and events simultaneously. Moreover, Node.js supports numerous frameworks and libraries essential for bot development.
One key characteristic of Node.js is its event-driven architecture, which allows real-time applications to operate smoothly. This is beneficial for handling messages instantly. Consequently, it has become the go-to choice for many developers wanting to create efficient Discord applications. However, it is important to note that while Node.js offers high performance, it requires a deeper understanding of asynchronous programming. This might be a hurdle for those new to coding.
Discord.js Library
Discord.js is a powerful library designed specifically to interact with Discord's API. It simplifies the process of creating bots by providing a set of user-friendly functions and methods that streamline development. Utilizing this library allows you to focus more on your bot's unique features rather than intricate API calls.
A notable feature of Discord.js is its intuitive object-oriented design. This makes it accessible for developers of various skill levels. It facilitates handling commands and events with ease. Given its popularity, a strong community support has developed around it, providing a wealth of resources and documentation. Disadvantages may arise from potential dependency issues or if the API updates create breaking changes in the library. Nevertheless, these instances are relatively rare and manageable.
Other Supportive Libraries
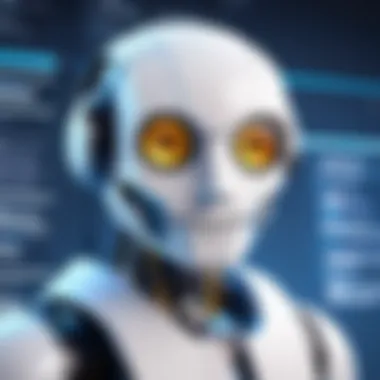
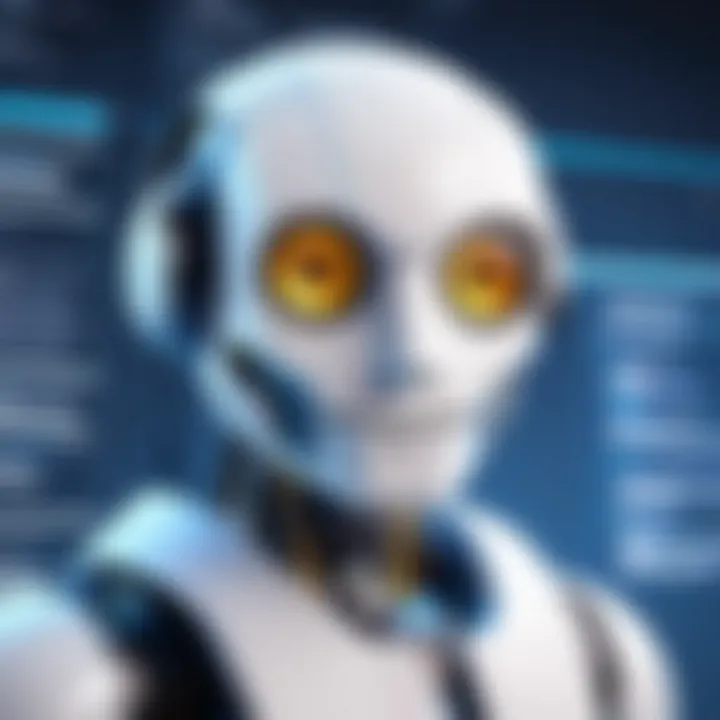
While Node.js and Discord.js are foundational, other supportive libraries can enhance your bot's functionalities. Libraries such as for making HTTP requests or for managing environment variables play critical roles in providing additional capabilities to your bot. They allow you to integrate third-party services, access APIs, and maintain secure configurations without cluttering your bot's codebase.
These supportive libraries are beneficial at different stages of development. For instance, integrating can help in adding a web interface for your bot. Furthermore, the ease of use of these libraries often means less time spent coding. However, it's essential to be cautious about the overhead they may introduce into your project, which could affect your bot's performance if not managed correctly.
Creating a Discord Application
Creating a Discord Application is the next logical step after setting up your environment. This process is where your bot begins to take shape in the Discord ecosystem. A Discord Application provides the necessary framework and identity for your bot, allowing it to interact with Discord servers.
To initiate this process, you will navigate to the Discord Developer Portal. Here, you can create your application, set its name, and configure its settings. This is a critical step as it generates the unique client ID and secret that your bot requires for functionality and security. Therefore, understanding this process is fundamental. Proper configuration can ensure that your bot operates correctly once deployed.
Programming Your Discord Bot
Programming a Discord bot is crucial as it defines its functionality and allows it to interact with users. Understanding how to write commands and responses is key to building a bot that enhances user experience. Additionally, it helps in managing automation tasks in a Discord server, improving community engagement. The process involves setting up basic structures, implementing commands, and listening to events.
Basic Bot Structure
A solid app structure forms the backbone of your bot. It generally involves organizing your files and code in a way that promotes scalability and maintainability. At its core, a basic bot structure will include a main file where your bot client starts running and additional modules for commands and events. By keeping your code modular, it's easier to read and debug.
- Main File: Contains the bot's initialization and configuration.
- Command Modules: Each command should have its own file, which makes adding new features simpler.
- Event Handlers: These manage user interactions like message sends and reactions.
Having a clear structure is beneficial as it allows teams to collaborate more effectively and lets new developers onboard quickly.
Implementing Commands
Implementing commands is about defining how your bot will respond to user input. This involves the following:
Command Structure
A command structure is a template for how your bot will receive and execute commands. This aspect is essential because it shapes the user experience significantly. A common practice is to define a prefix for your commands, allowing users to trigger them easily. For example, a bot might use as a prefix:
- Example Command:
- Response:
This straightforward command structure makes it easier for users to remember commands. It also allows for easy parsing and managing of command behavior. However, it’s important to handle user errors gracefully, providing feedback when an incorrect command is inputted.
How to Handle User Input
Handling user input is about processing the commands and arguments users send to the bot. The focus is on making the bot responsive to feedback and adaptable to different inputs. This typically requires:
- Input Validation: Check if the command is valid before executing it.
- Parameter Parsing: Extract arguments from the command string correctly.
A well-defined input handling mechanism reduces errors and enhances user satisfaction. It allows the bot to work smoothly even when unexpected inputs are presented.
Adding Event Listeners
Adding event listeners allows your bot to react to various interactions on Discord. It’s crucial for creating a dynamic and engaging experience for users. This includes listening for messages, user reactions, and other events to trigger appropriate responses.
Listening to Messages
Listening to messages is one of the primary functions of a Discord bot. When you set up your bot, you want to make sure it can respond to messages in channels. It’s often implemented using an event listener that triggers actions when a new message is received. For instance:
- Example: A bot responds with a welcome message when a new user joins a channel.
This functionality is essential because it not only fulfills user requests but also helps in community building. The challenge here lies in efficiently managing the event flow to prevent your bot from becoming overwhelmed, especially in busy servers.
Responding to Events
Responding to events involves defining how your bot reacts to different triggers. This can range from responding to user commands to automated reactions based on specific events within a server. The key characteristics of event responding are:
- Flexibility: The ability to program responses for a wide range of scenarios.
- Real-time Interaction: Ensuring the bot reacts quickly to events.
A unique feature of this approach is the ability to provide users with a personalized experience, engaging members in real-time activities. However, complexity can increase as the number of events handled grows, which may require stringent testing and careful planning.
Testing Your Bot Locally
Testing your Discord bot locally is a crucial step in the development process. It allows you to interact with your bot in a controlled environment, identify issues early, and ensure functionalities are working as intended before going live. This stage grants the developer the opportunity to make adjustments without affecting users, which can enhance the overall stability of the bot.
Running the Bot on Your Machine
To run your bot on your machine, you will need to ensure that you have set up your development environment correctly. With Node.js and Discord.js installed, it's time to execute your bot script. This process generally involves using a terminal or command prompt to navigate to your bot’s directory and running the command:
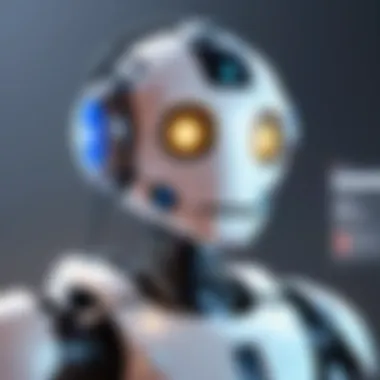
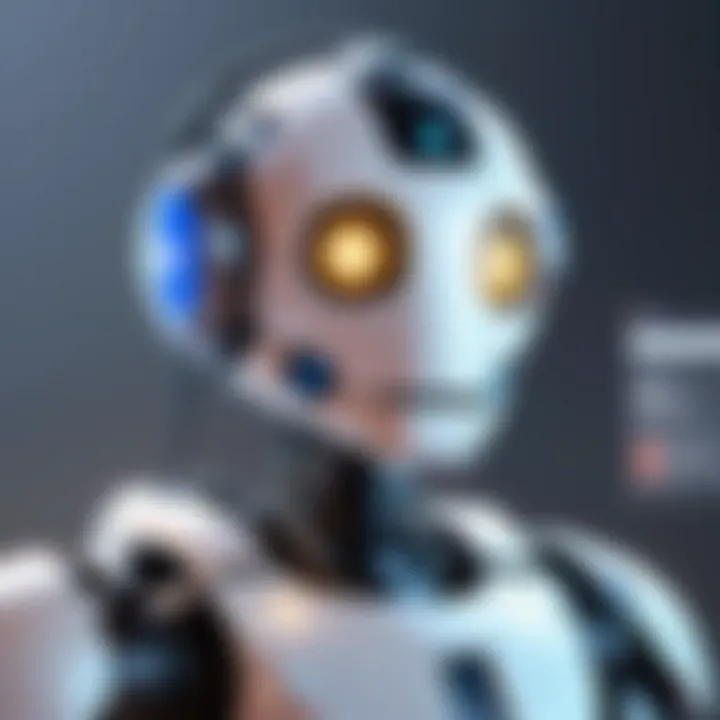
In this command, should be replaced with the name of your bot's main file. Once you execute this command, your bot should connect to the Discord API. It is important to watch the console output for any error messages that may indicate issues with the connection or coding errors in the script.
Debugging Common Issues
Despite thorough planning, issues may arise during testing. Understanding how to debug is vital for bot development. Here are a few common errors you might encounter, along with suggestions for resolving them:
- Missing Discord Token: If your bot fails to connect, check that the token in your script matches the one provided by the Discord Developer portal.
- Incorrect Permissions: Ensure that your bot has the correct permissions set in the Discord server settings. Lack of permissions can prevent the bot from performing certain actions.
- Code Errors: Pay attention to syntax errors or incorrectly structured code. Reading through the log output often highlights where the problem lies.
When troubleshooting, it might be helpful to use console prints in your code. This can help trace what part of the script is executing as expected and reveal where it's failing.
"Testing is not just an activity; it is a phase where you ensure the reliability and performance of your bot before sharing it with the world."
By adhering to these debugging practices, you can streamline the testing process and ensure that your bot is robust and ready for deployment.
Deploying Your Bot
Deploying your bot is a critical step in the development process. After you have programmed and tested your Discord bot, it is time to make it accessible to users. This process involves setting up your bot in a way that it can run continuously and respond to commands without interruption. Proper deployment ensures that your bot is reliable and efficient in serving the community.
There are various platforms available for hosting your bot. The choice of hosting can greatly influence performance, uptime, and the overall experience for users. Two primary options for hosting are cloud-based services and self-hosting solutions. Each has its own advantages and drawbacks, which we will discuss in detail.
Choosing a Hosting Platform
When selecting a hosting platform for your bot, consider factors like cost, ease of use, and scalability. The right service can enhance the functionality and accessibility of your bot. Below, we explore the two main types of hosting solutions: cloud options and self-hosting solutions.
Cloud Options
Cloud options are quite popular for deploying Discord bots. Providers like Amazon Web Services, Google Cloud, and Heroku offer robust infrastructure that can support high traffic and multiple users.
A key characteristic of cloud options is their scalability. As your bot grows in popularity, these services can easily accommodate increases in users and demands on resources. This allows for seamless performance.
A notable feature of cloud services is their managed hosting capabilities. This means that the hosting provider takes care of server maintenance, upgrades, and reliability issues. Users can focus more on bot development rather than dealing with technical server problems.
Self-Hosting Solutions
Self-hosting solutions allow for greater control over the environment in which your bot runs. By hosting on your own server or local machine, you have direct access to configuration and performance tuning.
A main characteristic of self-hosting is its cost-effectiveness. If you already own a server, this solution can save you from monthly cloud service fees. This approach is also suitable for those who want to experiment with bot features without the restrictions sometimes found in cloud hosting.
One unique feature of self-hosting is complete customization. You can choose the operating system, software stack, and any other parameters that align with your bot's requirements. However, this flexibility can come at a price. You are responsible for managing everything from security to server reliability, which requires technical know-how and can be time-consuming.
In summary, self-hosting can provide lower costs and customization, but it requires more effort and expertise.
Deployment Steps
Once you have chosen the appropriate hosting solution, the deployment process involves a few critical steps:
- Prepare Your Bot: Ensure your bot is tested and ready for deployment. This includes checking for bugs, optimizing code, and confirming that all features work as intended.
- Set Up the Server: Depending on your hosting choice, this might involve configuring your cloud environment or setting up your local server.
- Upload Your Code: Transfer your bot’s code to the server. For cloud hosting, this may involve using Git or FTP. For self-hosting, file transfer can be done using various methods, such as USB drives or network sharing.
- Launch Your Bot: Start your bot and monitor its performance. Depending on the platform, this might be through command line or the provider’s dashboard.
- Monitor and Optimize: After launching, keep track of the bot’s performance and make adjustments as necessary to optimize its efficiency and responsiveness.
By following these steps, you ensure a smooth deployment process and establish a reliable service for your Discord community.
Remember, the successful deployment of your bot is an ongoing process that involves regular monitoring and updates.
Inviting Your Bot to a Discord Server
Inviting your bot to a Discord server is a critical step in integrating its functionalities into the broader community context. Without this move, all the programming effort remains in isolation. The bot cannot serve its intended purpose or interact with users until it's added to a server. This section elucidates why this process is essential, explores the implications of making your bot accessible, and sets the stage for generating the necessary OAut URL.
One of the primary benefits of inviting your bot is enhancing engagement within the Discord server. By providing specific functionalities, such as game statistics or custom moderation, your bot can significantly improve user interactions. It enables automation of repetitive tasks, ensuring that server members can focus on more essential activities. Moreover, having your bot integrated into a server allows for real-time interaction, which is less achievable through standalone applications.
Generating the OAut URL
Generating the OAut URL is the key step in inviting your bot to a Discord server. This URL serves as the access point, effectively authorizing Discord to allow your bot to join. Without the OAut URL, the bot's capability to interact with any server remains nonexistent.
To create the OAut URL for your bot, you need to follow these steps:
- Navigate to the Discord Developer Portal: Visit the portal where your bots and applications are managed.
- Select Your Application: Locate the bot application that you have developed.
- Go to the OAut Tab: Here, you can find the necessary configurations.
- Set Scopes: Choose the scopes needed for your bot, such as , which specifically allows it to function as a bot.
- Select Permissions: You can define what permissions your bot requires. This step is crucial as it determines what actions the bot can perform.
- Copy the Generated URL: Once all options are configured, a unique OAut URL will be generated. Copy this URL.
This URL will be used to invite your bot to any Discord server where you have permissions to add bots.
Understanding Permissions and Scopes
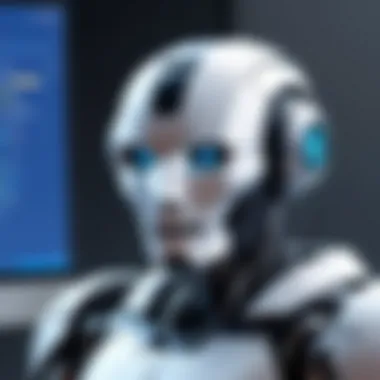
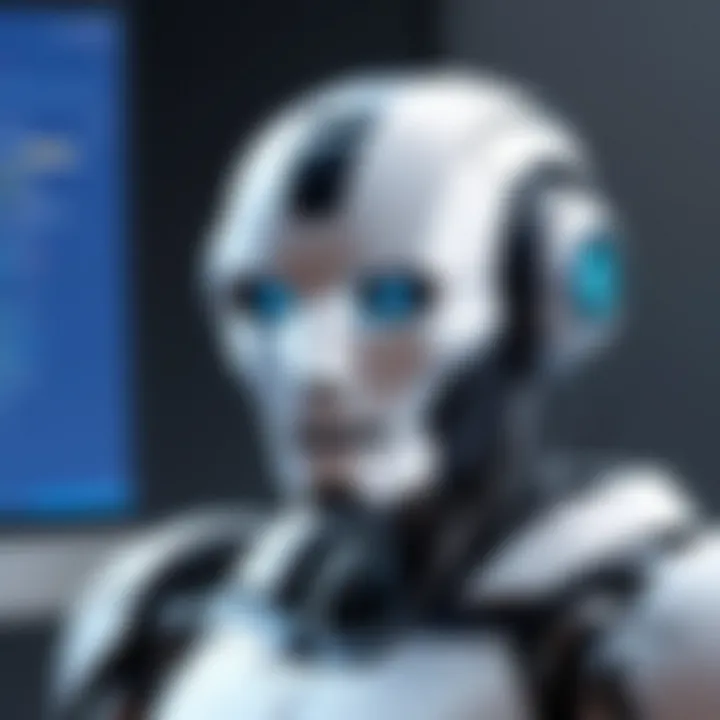
Understanding permissions and scopes is vital to ensuring that your bot operates effectively within a server's environment. Permissions dictate what your bot can do—whether it can send messages, manage channels, or ban users. Scopes, on the other hand, define the level of access your bot has when interacting with Discord's API.
When selecting permissions, consider the following points:
- Least Privilege Principle: Grant only the permissions necessary for your bot to function as intended. This enhances security and trust among server members.
- User Roles: Be mindful of how your permissions interact with the roles assigned to users in the server. Misconfigured permissions can lead to confusion or abuse.
- Testing Environment: It might be useful to test your bot in a personal server before deploying it to a larger community. This approach allows you to adjust permissions without affecting many users.
Common permissions include but are not limited to:
- : Lets your bot send messages in channels.
- : Allows your bot to create and manage channels.
- : Permits the bot to expel members from the server.
"Understanding the delicate balance of permissions and scopes will save you from future headaches as your bot scales. Always be cautious and deliberate with your choices."
Managing and Maintaining Your Bot
Managing and maintaining your bot is critical to its long-term success. After the initial setup and deployment, a bot will require ongoing attention to ensure it operates effectively. Users expect bots to be responsive and helpful. If a bot encounters problems frequently, it may lead to decreased user engagement and satisfaction. Therefore, careful management can enhance user experience and maintain your bot’s reputation.
Monitoring Bot Performance
Monitoring the performance of your Discord bot is crucial. This involves tracking various metrics to ensure optimal operation. Performance can be affected by several factors such as server response times, error rates, and user interactions. Here are some key points to consider:
- Response Time: Users expect near-instant responses. If your bot’s response time is slow, it reflects poorly on its functionality. Maintaining a low response time can improve user trust.
- Error Tracking: Implementing logging can help identify potential issues as they arise. Analyze error messages and adjust the code accordingly.
- User Engagement: By tracking how users interact with your bot, you can determine which features are popular and which are not. This could guide future updates.
To monitor these aspects, various tools and libraries can assist in collecting and analyzing data. Tools such as Discord.js can help log important events and metrics.
Updating and Upgrading Features
Technology evolves quickly, and so do user expectations. Keeping your bot updated with new features is important for maintaining relevance. This could involve enhancements or entirely new functionalities based on user feedback. Considerations for updating your bot include:
- Feature Requests: Engage with the user community to gather feedback. Listening to user needs helps steer development effectively.
- Bug Fixes: Users will encounter issues over time. Regularly review bug reports and prioritize them in updates.
- Security: Updating software libraries, especially those involving user data, is essential for security. Use alerts to stay informed about vulnerabilities in dependencies.
As you implement upgrades, document changes to allow users to understand new features or fixes. Having a changelog can keep users informed and build trust in your bot.
Regular updates are not only about fixing bugs but also about enhancing the overall user experience.
In summary, effectively managing and maintaining your Discord bot involves a combined approach of monitoring performance and consistently updating features. By focusing on these areas, you can ensure your bot remains functional, appealing, and secure for its users.
Common Issues and Troubleshooting
Common issues can arise when developing and deploying Discord bots. It is critical to understand these issues to ensure the bot operates smoothly. Troubleshooting is not merely about fixing errors but proactively identifying potential failures before they disrupt the user experience. Addressing these common issues increases the reliability of your bot and enhances user engagement.
Common Error Messages
During the creation and use of a Discord bot, one might encounter various error messages. Recognizing these messages can significantly aid in resolving issues quickly. Some frequent errors include:
- Invalid Token: If your bot is unable to connect, it may be due to an incorrect token. Double-check it in the Discord Developer Portal.
- Lack of Permissions: If the bot commands fail, it could indicate missing permissions within the server roles. Ensure you assign the appropriate permissions in the bot settings.
- Rate Limit Exceeded: Discord enforces rate limits on API calls. If your bot is making too many requests in a short period, it may be temporarily blocked.
Identifying and understanding these errors can save substantial time. Make a habit of checking Discord’s documentation for updates related to error codes and recommended fixes.
"Understanding and resolving common error messages is crucial to maintaining a functional Discord bot."
Finding Support Resources
Sufficient resources play an essential role in resolving issues. Many platforms offer assistance, guides, and community support. Here are some effective ways to find help:
- Discord Developer Portal: Provides extensive documentation about API usage and error handling.
- GitHub Repositories: Many projects are open source. Reviewing these can provide insights into solving specific problems you may face.
- Developer Communities: Platforms like Reddit and specialized Discord servers can be invaluable. Engaging with these communities offers multiple perspectives and solutions to similar issues.
Utilizing these resources enhances one’s skills in troubleshooting and can vastly improve the functionality and reliability of your bot. Keeping a list of useful links for quick access can streamline the process as well.
The End
In this section, we will summarize the significance of having a custom Discord bot and how it contributes to enhancing user engagement and community interaction. Creating a Discord bot is not just about programming; it provides a personalized experience for users. Automating tasks allows server administrators to focus on community building rather than mundane responsibilities.
Recap of Key Steps
As you conclude your journey in bot development, it is essential to recall the critical milestones covered in this guide. Here’s a brief breakdown of the key steps:
- Understanding Discord Bots: Get familiar with what bots are and how they can elevate communication in your server.
- Setting Up the Development Environment: Install necessary tools and create your Discord application.
- Programming Your Bot: Understand the basic structure and implement commands along with event listeners.
- Testing Locally: Run your bot and debug issues as they arise.
- Deployment: Choose the right hosting platform and complete the deployment steps.
- Managing the Bot: Regularly monitor performance and update its features to match user needs.
- Troubleshooting: Familiarize yourself with common errors and seek support when needed.
This recap solidifies the foundation built throughout the article, ensuring you have a clear path for development and maintenance.
Next Steps in Bot Development
Having established a functioning Discord bot, you may be wondering what comes next. Here are some recommended next steps to further enhance your bot's capabilities:
- Explore Advanced Features: Learn about interactive features such as reactions, buttons, and modals that can enhance user interaction.
- Utilize Third-Party APIs: Integrate external services to provide additional functionalities, such as games, weather updates, or music playback.
- Join Developer Communities: Participate in forums such as Reddit or Discord developer servers to share experiences and learn from others. This can foster growth and creativity.
- Regular Updates: Keep up with Discord’s API changes and adjust your bot accordingly to ensure functionality over time.
Each of these steps aims to expand your bot's potential and enrich user interaction. The future of your bot lies in continuous improvement and adaptation to user feedback and needs.