Creating a Discord Bot with JavaScript: A Complete Guide
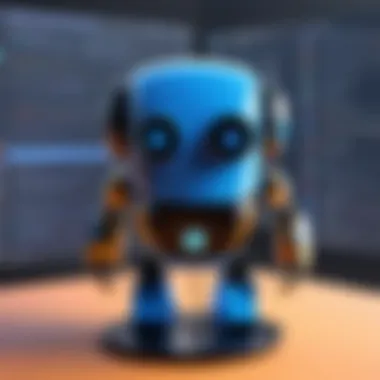
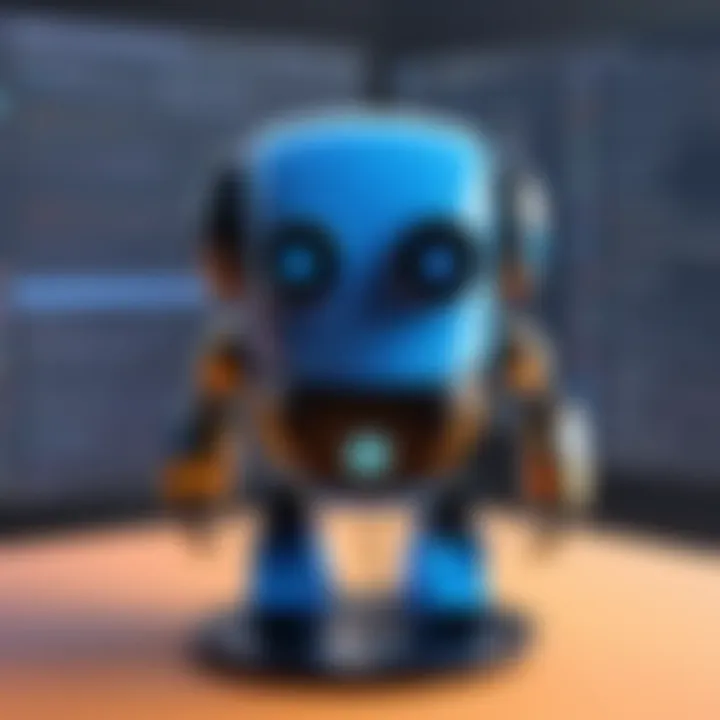
Intro
In recent years, the surge in popularity of Discord as a communication platform has led to a growing interest in creating bots. Bots automate processes and enhance user engagement within servers. Developing a Discord bot with JavaScript allows you to harness its functionality and customize interactions. This article guides readers through the essential components of bot development, focusing on the best practices and potential hurdles one may encounter along the way.
Creating a bot is not merely about writing code; it involves understanding Discord's unique environment and API capabilities. This article will provide insights into the prerequisites, development techniques, and various deployment methods available for JavaScript enthusiasts. By integrating practical examples and strategies, readers will gain knowledge applicable to their own projects in Discord's expansive ecosystem.
Whether you're a beginner eager to explore or a seasoned developer wishing to refine your skills, this guide will act as your companion in navigating the complexities of bot creation. The focus will be on practicality and relevance, ensuring that the information is accessible yet thorough.
Preface to Discord Bots
Discord bots play a pivotal role in the functionality and interactivity of Discord servers. Understanding what discord bots are and why they are created is crucial for anyone looking to enhance their Discord experience or streamline server management. This section will unravel the fundamental concepts of Discord bots, dive into their definitions, and illustrate their purposes within the ecosystem of Discord.
Bots are essentially automated programs that can perform tasks and respond to events on servers without human intervention. They can manage conversations, play music, moderate content, or interact with members. The importance of these bots extends beyond mere convenience; they serve to improve user engagement, automate repetitive tasks, and maintain order in servers that house large communities.
As a developer or enthusiast delving into bot creation, establishing a solid foundation begins with comprehending the purpose they serve in Discord. This knowledge will steer the development process effectively, ensuring that the bots created align with the intended functionalities and ultimately enrich the user experience.
Definition and Purpose
A Discord bot can be defined as a non-human user that performs automated tasks on Discord. They operate by responding to commands issued by server members, executing a variety of functions based on the code programmed into them. The purpose of these bots varies significantly, reflecting the different needs of Discord communities.
Some common functions of Discord bots include:
- Moderation: Managing server rules by responding to inappropriate content or spam.
- Games and Entertainment: Offering interactive games or music playback to make servers more enjoyable.
- Informational: Providing server members with information like news or statistics on different topics.
In essence, the purpose of Discord bots is to enhance server interactivity and user experience. As the user base of Discord continues to expand, the demand for customizable and efficient bots has grown. This trend showcases the value of bots in fostering community and engagement among users.
Overview of the Discord API
The Discord API is the backbone that allows developers to create, manage, and control Discord bots. It offers a set of tools that interface directly with Discord's services. Using the API, developers can connect their bots to servers, send messages, and manage various functionalities dynamically.
The API operates based on WebSocket connections, allowing for real-time interaction with Discord. This design not only enhances performance but also provides a seamless experience for users engaging with bots. Here are some key components:
- Endpoints: The API consists of various endpoints to manage users, messages, channels, etc.
- WebSockets: Utilize WebSockets for real-time event handling, enabling immediate responses to user actions.
- Rate Limits: Developers should be aware of rate limits imposed by Discord to prevent overwhelming the servers with requests.
Understanding the Discord API is essential for anyone venturing into bot creation. It not only facilitates the overall development process but also helps in creating more effective and responsive bots for varied Discord communities.
Prerequisites for Development
Understanding the prerequisites for developing a Discord bot is essential for a seamless experience. These elements lay the groundwork needed to create a bot efficiently. Without these, you might face setbacks that could derail your progress and create frustration.
Understanding your tools and technology can make the difference between a smooth development process and facing many roadblocks. The right tools help streamline your workflow, while foundational knowledge in programming allows you to navigate challenges effectively. We will focus on two main aspects: necessary tools and understanding of JavaScript basics.
Necessary Tools
Node.js Installation
Node.js is crucial for building Discord bots. It allows for running JavaScript on the server side. The ability to run JavaScript outside a browser makes it a powerful choice for server applications, including Discord bots.
One key characteristic of Node.js is its use of an event-driven, non-blocking I/O model. This makes it efficient for handling multiple connections simultaneously, which is significant for Discord’s real-time messaging environment. It provides great performance and scalability, appealing traits for bot development.
Using Node.js can introduce you to npm (Node Package Manager), which provides a vast repository of libraries. This feature enables easy installation of packages that can extend your bot's capabilities. However, one should be aware that Node.js requires a basic understanding of its environment and JavaScript. Without this familiarity, you may find it challenging initially.
Text Editor Options
Selecting the right text editor can influence your development speed and comfort. Popular options like Visual Studio Code, Atom, and Sublime Text provide features like syntax highlighting and code completion, making coding easier and more organized.
Visual Studio Code is considered a favorite due to its integrated terminal and support for various extensions. Its functionality improves the coding experience by providing tools that help you write and manage code efficiently.
However, the choice of text editor is subjective. Some developers may prefer lighter editors for their simplicity. The unique feature is often user preference, as each editor has advantages and disadvantages in workflow and performance.
Understanding JavaScript Basics
Essential Syntax
JavaScript's syntax forms the backbone of your code. Getting a solid grip on the essential syntax is vital as it aids in creating functional and error-free code. Key topics include variable declarations, data types, functions, and control structures, among others.
The straightforward syntax helps make JavaScript beginner-friendly while also supporting advanced programming techniques. The significant advantage is its flexibility in coding styles. However, this flexibility may also lead to less structured code if not handled correctly. Adhering to best practices can mitigate this issue.
Common Functions
Familiarity with common JavaScript functions enhances your ability to implement features effectively in your bot. Knowing built-in functions like , , and can save time in handling arrays and objects. These functions represent powerful tools for data manipulation.
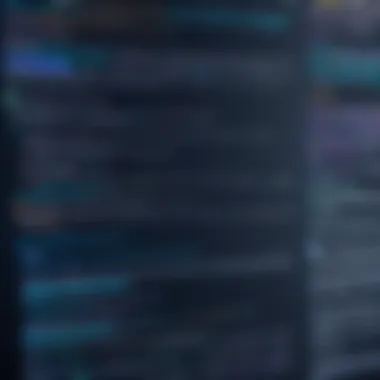
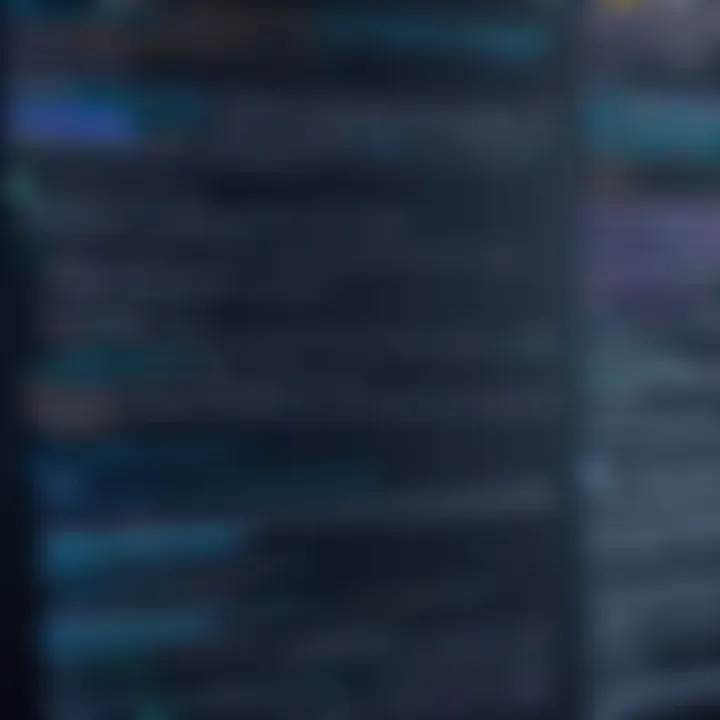
The advantage of using common functions lies in increasing code efficiency and reducing the need to write repetitive code. They offer reusable logic, which is beneficial in maintaining your bot’s functionality over time. Yet, relying solely on them might lead to underestimating other important programming concepts that are equally essential.
Bot development requires a balanced skillset that combines a solid foundation in tools with practical coding knowledge. Stay committed to learning and experimenting.
Creating a New Discord Bot
Creating a Discord bot is a pivotal step in developing the automation tools and features that can enhance user experiences within servers. This section delves into the processes involved in creating a new bot, from initial application setup to obtaining a bot token. Each element plays a crucial role in ensuring the bot functions effectively and securely.
Setting Up a Discord Application
Accessing the Developer Portal
Accessing the Developer Portal is the first action a developer must take when starting the bot creation process. This portal serves as the hub for app development on Discord, providing the tools necessary for bot administration. A distinct feature of the Developer Portal is its user-friendly interface, which simplifies the navigation process, making it ideal for both novice and experienced developers.
The importance of this portal cannot be understated. It not only allows developers to create their applications but also to manage their settings, enabling or disabling bot functionalities. A key characteristic is its integrated support for bot-related documentation, which greatly enhances user comprehension. However, users must keep in mind that security rules apply, and one must adhere to these guidelines to prevent account suspension or inconvenience.
"Accessing the Developer Portal is the gateway to all functionalities and features offered by Discord for bot developers."
Creating a New Application
Following access to the Developer Portal, the next step is creating a new application. This acts as a blueprint for the bot itself. When creating this application, developers can input key information such as the application name and description. A notable aspect of creating a new application is the ability to customize application settings. This is beneficial for ensuring that users understand what the bot is intended to do.
The unique feature of this process includes setting various scopes and permissions, which define the bot's capabilities in a server environment. Understanding this is vital for future interactions with Discord’s API. However, some developers may find it challenging to determine the appropriate permissions needed, potentially leading to functionality issues later on.
Generating a Bot Token
Understanding Token Security
Generating a bot token is another critical aspect of bot creation. The token acts as a password that allows the bot to authenticate itself with Discord's servers. Understanding token security is essential as it ensures that the bot operates within a secure framework. A defining characteristic of token security is the recommendation against sharing the token publicly or allowing it to be exposed in any way.
The token also includes specific features that enhance security, such as the ability to revoke it if compromised. Proper management of a bot token can hinder potential security breaches and unauthorized access, making it a significant focus in this article.
Storing Tokens Safely
Once the bot token is generated, the next consideration is storing tokens safely. This step is critical for maintaining the integrity and security of the bot. Developers should utilize secure storage practices, such as environment variables or configuration files that are excluded from version control. This prevents exposure of the token in public repositories.
A key characteristic of safe storage practices is the reduction of risk. This practice ensures that even if unauthorized users gain access to project files, they do not access sensitive information needed to control the bot. However, this approach comes with its own complexity, as developers must set up this storage system effectively.
By understanding these processes, developers will be better equipped to create a Discord bot with strong functionality and security measures in place.
Developing the Bot Functionality
Creating a fully functional Discord bot goes beyond initial setup. This stage, Developing the Bot Functionality, focuses on crafting engaging user experiences through various automated processes. Understanding how to effectively implement features is crucial as it not only enhances interactions but also meets the specific needs of the server users.
The primary elements covered here include setting up the project structure and utilizing libraries like Discord.js. Each aspect helps ensure that the bot runs smoothly and serves its intended purpose.
Setting Up the Project Structure
Setting a well-defined project structure is essential for efficient development. It allows for organized code management, which is significant when your bot becomes more complex. A clean directory setup helps developers navigate their files easily, making debugging simple and updates manageable.
Directory Setup
Creating a logical directory structure is the first step towards a well-organized project.
A standard setup might include directories for commands, events, and configurations. This organization makes it easier to locate files related to specific functionalities. For example, having a dedicated folder for commands helps to isolate command functionalities from event handling.
The key characteristic of a good directory setup is clarity. Developers can quickly understand where files live. This transparency becomes beneficial during collaboration or when revisiting the project after a break.
However, a potential downside may arise if the project grows uncontrollably. An overly complex structure can lead to confusion. Therefore, always aim to keep it simple and relevant to the project’s scope.
Package Management with npm
Using npm (Node Package Manager) is a common choice for managing packages in JavaScript projects. This tool simplifies the process of installing, updating, and managing dependencies required for the bot.
The primary advantage of npm is its extensive registry of libraries and tools. This gives developers access to countless resources that can enhance their bot easily. For instance, libraries like dotenv for handling environment variables are just a few commands away.
However, using npm comes with considerations. Regularly updating dependencies is necessary to avoid security vulnerabilities. While npm does provide a straightforward way to manage packages, it sometimes leads to dependency conflicts that can prove challenging to resolve.
Using Discord.js Library
Discord.js is a powerful library widely used for building Discord bots. Its rich feature set simplifies many tasks that would otherwise be complex to implement within the bot. By leveraging this library, developers can interact with the Discord API effectively.
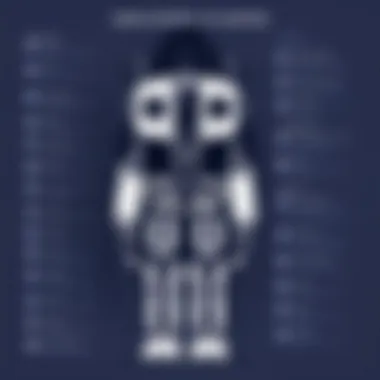
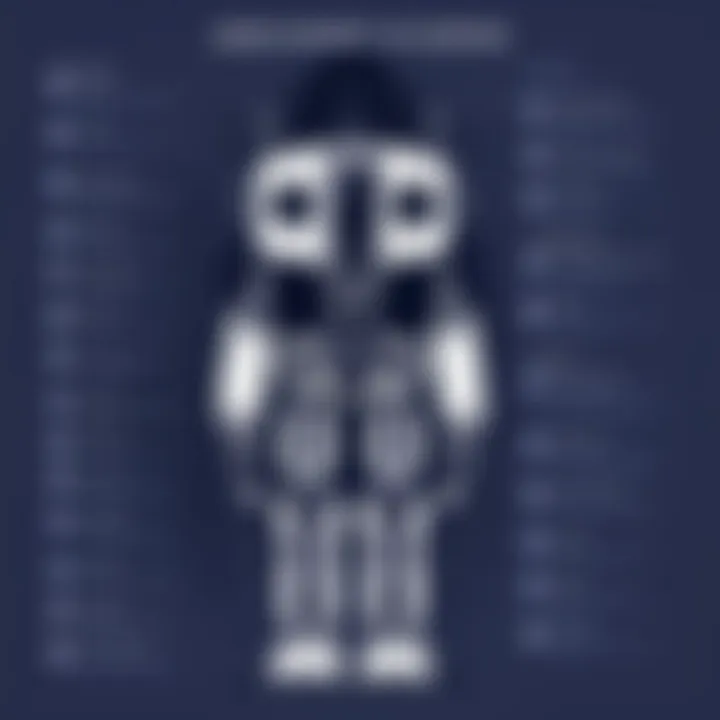
Installation and Setup
The installation process for Discord.js is a simple but essential step in bot development. It typically involves running a command in the terminal.
This direct installation from npm allows for quick setup and immediate access to the library’s functions. The ease of installation helps developers to get started without unnecessary delays.
A unique feature of Discord.js is its support for both JavaScript and TypeScript, offering flexibility for developers working in different contexts. While it generally works well, there have been instances where new updates introduce breaking changes, requiring developers to be cautious.
Basic Commands Implementation
Once Discord.js is set up, the next focus is implementing basic command functionalities. This is where the bot starts engaging with users directly.
Basic commands can range from simple text responses to executing more complex tasks. This range offers developers a chance to explore their creativity in how users interact with their bot.
The distinctive advantage of implementing commands early on is allowing for immediate user feedback. Testing commands in real-time helps refine their functionality and discover any potential issues.
However, it is crucial to ensure commands are designed with clarity. Poorly structured commands can lead to confusion among users, affecting their overall engagement with the bot. Keeping commands intuitive will enhance user experience significantly.
Remember that the effectiveness of your bot heavily relies on the implemented functions and their responsiveness.
In summary, Developing the Bot Functionality sets the stage for a successful Discord bot. Structured organization, right libraries, and thoughtful command implementation are key in creating a robust environment for user interaction.
Implementing Advanced Features
Implementing advanced features is crucial in the development of a Discord bot. These functionalities enhance the user experience and allow for greater interaction within the community. Focusing on event handling and command structures forms a strong basis for a bot’s performance. This section covers how to listen to user messages, respond to events, and manage commands and permissions. These elements are vital for creating engaging and responsive bots that can execute complex tasks and cater to user needs effectively.
Event Handling
Event handling is a core aspect of bot functionality. It allows the bot to react to various activities and events occurring on the server. This includes user interactions like messages sent, members joining or leaving, and even reactions to messages. Effective event handling improves user engagement and keeps the server lively.
Listening to User Messages
Listening to user messages is a foundational functionality for any Discord bot. This feature enables the bot to receive and process input from users, making it possible to carry out commands and provide information on demand. The primary characteristic of listening to messages is its ability to facilitate real-time communication within the server. This immediacy is a significant benefit, as it allows users to interact naturally with the bot without delays.
Moreover, this feature can be tailored to respond to specific commands embedded in user messages. The unique aspect of listening to messages is its adaptability; you can configure the bot to recognize a variety of keywords or phrases that trigger certain responses. However, one must be cautious to avoid overloading the bot with too many commands, which could lead to confusion or slow performance.
Responding to Events
Responding to events represents another important feature of advanced bot development. This involves taking action based on various triggers, such as user interactions or server changes. The key characteristic here is its dynamic nature—the bot can be programmed to perform multiple tasks automatically when certain conditions are met. This autonomy greatly enhances the bot's utility and is why it is often a sought-after feature.
A unique feature of responding to events is the bot's capability to engage proactively with users. For example, a bot could welcome new members or notify users about events. This responsiveness fosters a more inviting environment. However, developers should consider the potential for spam if the bot reacts too frequently or inappropriately, which could erode user experience.
Adding Commands and Permissions
Adding commands and permissions is essential for allowing users to interact with the bot in a controlled manner. This structure organizes how commands are executed and determines who can do what within the server, enhancing both functionality and security. The openness of command execution can lead to varied interactions, which is beneficial for user engagement.
Command Structure
The command structure lays out how the bot interprets and executes user input. It plays a significant role in ensuring that commands are consistent and efficient. A well-defined command structure is beneficial as it makes it easier for users to understand how to interact with the bot. One key characteristic is the ability to establish prefixes or triggers that identify commands within messages.
This structure makes the bot accessible even for new users, as clarity in commands helps prevent misunderstandings. A unique feature within command structure can be the inclusion of different parameters, allowing for varied responses based on user input. On the downside, an overly complex command layout may confuse users, so balancing simplicity and functionality is critical.
Role-Based Access Control
Role-Based Access Control (RBAC) is a method that enhances command execution safety within the bot's framework. By implementing RBAC, developers can define who has permission to use specific commands. This contributes to better management of the server's interactions and ensures that sensitive commands are restricted to designated users only.
The key characteristic of RBAC is its granularity, allowing for precise control over user access levels. This is beneficial in larger servers where different users hold varying responsibilities. A unique feature of RBAC is the ability to manage permissions dynamically, meaning roles can be adjusted as servers evolve. One disadvantage is the potential for administrative overhead; maintaining roles and permissions can become cumbersome if not managed properly.
Testing and Debugging
Testing and debugging are crucial phases in the development of a Discord bot. These processes ensure that the bot operates as intended, without errors or bugs that can disrupt user experience. Proper testing catches flaws early, saving time and effort in the long run. Debugging helps to identify and fix problems more efficiently.
The benefits of rigorous testing are manifold. First, it enhances the reliability of the bot, which is important in maintaining user trust. A bot with frequent errors can drive users away. Second, effective debugging reduces downtime, enabling smoother server interactions as the bot performs its tasks. Considerations include determining what to test, how to test, and weighing the use of various strategies.
Debugging Strategies
Using Console Logs
Using console logs is a fundamental technique in debugging for a Discord bot. Console logs help track the flow of the code and identify where things might be going wrong. Developers insert logging statements throughout their code to output the current state or values of variables at critical execution points.
This method is benefiting because it is straightforward and requires minimal setup. The key characteristic of console logging lies in its immediate feedback. It allows developers to see real-time data or errors as they occur. However, excessive logging can clutter the output, making it harder to find valuable information. Keep logs concise to maintain clarity.
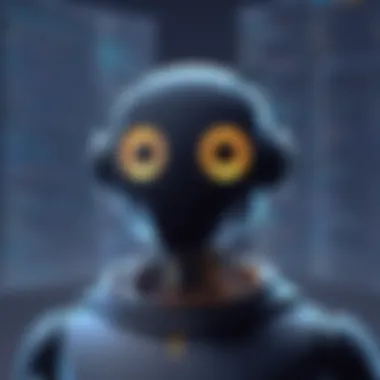
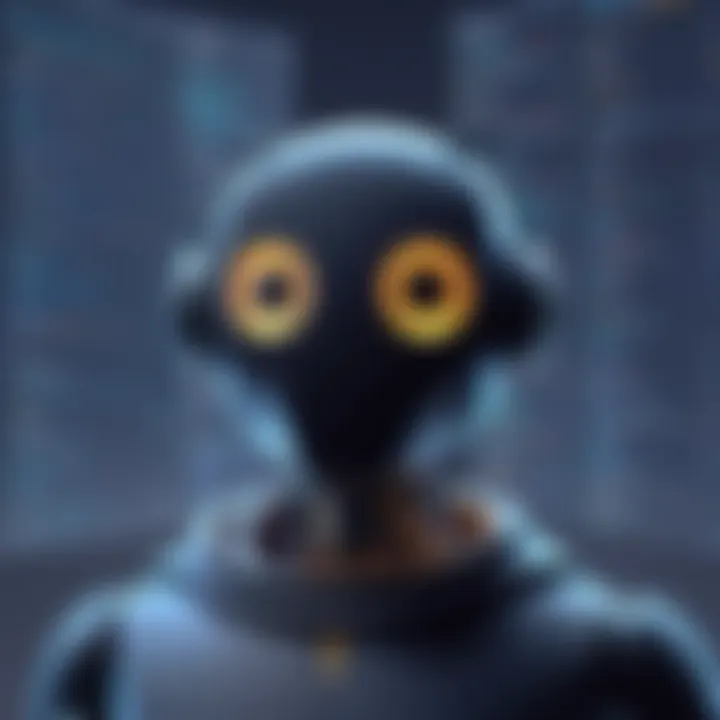
Error Handling Techniques
Error handling techniques are essential for managing exceptions and ensuring that the bot remains functional even when issues arise. These techniques allow developers to anticipate potential errors and respond appropriately, enhancing the overall reliability of the bot.
This approach is popular because it prevents unhandled exceptions from crashing the bot. The unique feature of effective error handling is the use of try-catch blocks. This method allows developers to catch errors and handle them gracefully, often by providing user-friendly error messages or logging the issue for later fixing. However, over-relying on catch blocks can lead to poor performance if not managed properly.
Testing Bot Functionality
Testing bot functionality involves ensuring that all features operate as intended. This phase can often reveal areas needing improvement or additional capabilities.
Creating Test Scenarios
Creating test scenarios is a structured way to evaluate a Discord bot's performance and reliability. Test scenarios outline specific conditions or inputs that the bot must handle, providing a framework for validation.
The key characteristic of crafting effective test scenarios is specificity. Well-defined scenarios cover diverse cases, from normal to edge cases. They help ensure that the bot can handle unexpected inputs and conditions. One advantage is that it leads to more comprehensive testing; however, the downside is that creating these scenarios can be time-intensive.
Validating Output
Validating output focuses on checking that the results produced by the bot meet the expected outcomes. This aspect of testing checks both functionality and the accuracy of the responses generated during bot interactions.
Validating output is a beneficial choice for ensuring user satisfaction and trust. The unique feature of this practice lies in the consistency it provides; by applying standardized response checks, developers confirm that the bot behaves reliably. The challenge here is managing the variety of possible user inputs, which can complicate validation efforts.
Deploying Your Discord Bot
Deployment is a critical stage in the development of a Discord bot. It is not enough to create a bot; it must also run reliably and efficiently in a live environment. Deployment ensures that your bot can interact with users and respond to their requests in real time. The right deployment choice can affect the performance, uptime, and user experience of the bot.
Hosting Options
Local vs. Remote Hosting
When considering hosting options, developers need to choose between local and remote hosting. Local hosting involves running the bot on a personal machine. It's relatively simple to set up and allows full control over the environment. However, this option has limitations. If the developer's machine goes offline or experiences issues, the bot becomes unavailable. On the other hand, remote hosting provides the advantage of continuous availability. It allows the bot to run on dedicated servers, ensuring better uptime and performance. This makes remote hosting a more popular choice for deploying bots that require constant interaction with users.
Each option has unique features worth noting. Local hosting is often more suited for testing purposes or casual use, while remote hosting accommodates scalability and better resource management.
Popular Hosting Platforms
There are various popular hosting platforms to consider for deploying Discord bots. Services such as Heroku, DigitalOcean, and AWS EC2 are widely recognized for their reliability and scalability. Each platform offers distinct advantages that cater to different needs. For example, Heroku provides a user-friendly interface for beginners and allows easy integration with version control systems. This characteristic makes it an accessible choice for new developers. However, it may present limitations regarding scaling for complex bots.
In contrast, platforms like AWS offer extensive scalability options. They can handle larger loads, but require a deeper understanding of cloud services. Moreover, there can be a steeper learning curve for setup compared to more beginner-friendly options.
Bot Maintenance and Updates
Proper maintenance is essential for a Discord bot to function effectively over time. Neglecting maintenance may lead to issues that diminish the bot's performance or usability. Regular checks and updates are key to maintaining efficiency.
Routine Checks
Routine checks focus on monitoring the bot's performance and identifying potential problems before they escalate. Regular inspections can highlight any irregular behaviors or errors. This is particularly important in a production environment where uptime is crucial. One key aspect of routine checks is performance monitoring. By keeping an eye on memory usage, response times, and error rates, developers can gather insights into how the bot is functioning overall. Automated alerts can be implemented for immediate action when performance thresholds are breached. Regular checks ensure that the bot continues to offer a positive user experience.
Updating Dependencies
Dependencies play a vital role in the functioning of a Discord bot. Over time, libraries and frameworks used within the bot may receive updates that introduce new features or security patches. Updating dependencies regularly is crucial to leverage these improvements. Failing to update may leave the bot vulnerable to bugs or security issues. This characteristic makes dependency management a must for developers who want their bots to remain secure.
Additionally, frequent updates can improve performance and add new functionalities to the bot, keeping it relevant in a fast-paced environment like Discord. While the process requires diligence, the advantages are significant when it comes to enhancing user experiences and maintaining security.
The End
In this article, the conclusion serves as a pivotal segment, summarizing the key elements discussed throughout the guide on creating a Discord bot using JavaScript. It highlights the importance of understanding both foundational concepts and advanced features that have been covered. The journey from initial setup to deployment encapsulates a broad spectrum of knowledge necessary for aspiring developers.
The benefits of implementing a Discord bot are manifold. They enhance server functionality through automation, allowing for the management of member interactions and content delivery. Considerations are also paramount; developers must prioritize security, especially when handling sensitive tokens and bot permissions. The feedback loop from users can provide invaluable insights into improving bot features and user engagement.
Additionally, the exploration of hosting options and ongoing maintenance signifies that bot development is not merely a one-time event but an ongoing process. This necessitates continuous learning and adaptation in response to updates in the Discord API and user needs.
"The best Discord bots evolve with their communities, offering tailored functionalities that enrich the user experience."
The culmination of this article reinforces the relevance of not only technical skills but also the understanding of community engagement and user experience design in bot development.
Key Takeaways
- Understanding the Discord API: Familiarity with the Discord API is essential for creating responsive and effective bots.
- Security Practices: Always prioritize security, especially with bot tokens and permissions, to protect user data and bot integrity.
- Continuous Maintenance: Regular updates and maintenance are crucial for ensuring smooth operation and utilizing new features.
- Community Engagement: Being attuned to user feedback can enhance bot relevance and improve overall functionality.
- Embracing Challenges: Encountering challenges during development is part of the learning process; solving these will fortify your skill set.
Future Directions for Bot Development
The landscape of bot development is always evolving, particularly on platforms like Discord. Looking ahead, several trends may impact how developers approach their projects:
- Integration with AI: As artificial intelligence continues to advance, leveraging AI for smarter automation can significantly enhance bot capabilities, making interactions more natural and engaging.
- Enhanced User Experience: Developers are increasingly focusing on UX design in bot functionality, ensuring intuitive interactions.
- Improved Event Handling: With the rise of more complex user activities, developing sophisticated event handling will be imperative to respond dynamically.
- Cross-Platform Functionality: As chat applications diversify, building bots that can interface across multiple platforms may be a valuable direction.
The future will require developers to be adaptable, embrace new technologies, and maintain a commitment to user-focused development. Continuing to learn and experiment within this field will ultimately yield more innovative and powerful Discord bots.